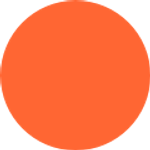
Krisztian Kovacs
Published on 30 April 2018
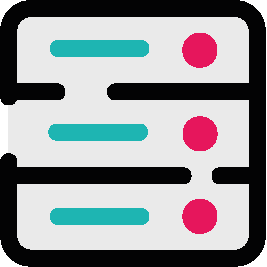
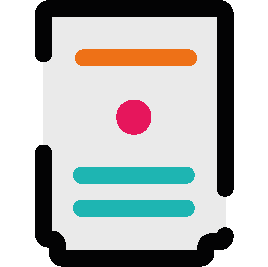
Five tips for writing cleaner code
In this post, we go back to basics with a guide to writing clean code. We'll explain why it's important to write code in a way that anybody can understand it first-time.
In this post, we're going back to basics with a guide to writing clean code. We'll explain why it's important to write code in such a way that anybody can understand it first-time.
So let's start with why writing clean code is so important.
One of the best handbooks I have read on this topic is the aptly named Clean Code. The author, industry expert, Robert C Martin suggests that "Even bad code can function. But if the code isn’t clean, it can bring a development organisation to its knees". Martin's perspective here gives you an idea of the role that clean code can play in making or breaking a project.
For me, writing code is a lot like writing a blog. In both cases, you have to research and decide what you want to write and what you want your writing to achieve. In the same way as creating a blog, when you write code, you have to structure your thoughts in a way that other people will understand and will be able to follow logically.
1. Make your code readable
When I write code I always keep in mind that there is a 100% chance that I will need to revisit it again in the future. This is true for most developers who work on multiple projects, once you have created code you may not get a chance to look at it again for weeks.
Early in my career, I used to write code in one big chunk. I used values such as a, b, tsm, smb, etc. Though it made perfect sense to use these values at the time, fast forward a few weeks and I would have no idea what I meant by 'tsm'. If I couldn't remember what my code was, how could anyone else?
To help remind me of what I wrote and why I wrote it, I soon realised that I needed to make my code readable, so my value 'tsm' became 'timeSettingManager' and so on. I also divided the code into small manageable pieces with a logical structure so that anyone could follow it.
2. Make your code reusable
To make my code reusable, I use what I call ‘code minions.’ Minions are a fictional species that are loyal and resilient, and there are millions of them. The same applies to my code minions.
Let me provide an example. I want to use ScriptRunner for Jira to retrieve data on software issues. By utilising my army of code minions I can copy one I created previously and paste it into my script.
Here is an example of a code minion in action:
static getCustomField(Issue issue, String customFieldID) {
return issue.getCustomFieldValue(ComponentAccessor.getCustomFieldManager()
.getCustomFieldObject(customFieldID))
}
And here's how I reference it:
CustomField myLittleCustomField = getCustomField(anIssue, "customfieldid")
The idea is to have all my code minions stored and categorised, ready to be used when I need them. Following this method ensures one essential thing - I never break my code by pasting extra lines.
3. Make your code easy-to-use and share
Clients often use the code I create, and ask me to develop it in such a way that it is easy to install in their environment. To make it simple for them to follow and use, I create a header for all my scripts, that looks like this:
//-------------------------------------------------------------------------
// This script will display a warning message if the date is between
// the start and end date of the issues filtered by the given query.
// The script is universal in the sense that it can be added to any
// date field and will display the warning under it (in the description).
//-------------------------------------------------------------------------
// Please change the following so the script would suit your needs:
String changeFreezeStartDateField = "customfield_10300"
String changeFreezeEndDateField = "customfield_10301"
String jiraAdmin = "admin"
String filterQuery = "Freeze Period"
String fieldNameOnScreen = "Change Start Date / Time"
//-------------------------------------------------------------------------
So instead of the client having to alter the code at lines 12, 187, 299, 305 and so on, they can do any necessary modifications to the header.
4. Make your code easy-to-view
I usually create my code in portrait mode using a full HD (High Definition) monitor, that means instead of having a resolution of 1920x1080, it is 1080x1920.
People use different computers, devices, and monitors to view code so to avoid any code breaks on smaller screens, it is vital that I keep my lines of code less than 80 characters long. Following this rule is particularly helpful when I am checking my code for bugs or issues via ‘screen share’ on client machines.
As we often spend more time reading code than writing it, using good naming conventions for your code will make your life easier. Using clean code naming conventions (long, descriptive names) I can store my discoveries in my code. For example, I once figured out how to extract specific dates from Jira:
Date getFieldDate( String fieldName) {
def formField = getFieldByName(fieldName)
String stringDate = formField.getValue()
Date date = new Date().parse("EEE MMM dd kk:mm:ss z yyyy", stringDate)
return date
}
Parsing the date is a somewhat tedious process as the goal is to create a solution that enables one date to compare with another date. Figuring out this process and recording the discoveries within my code is very helpful, in this case, I ended up using the code in four other scripts created a few days later.
Again, if I take this code and paste it into any script, it doesn't do anything until I call it.
if (issue.created == getFieldDate("Start Date")) dostuff...
The above example shows how one could compare the parsed date with a system field date in Jira. As the two date formats are the same, it will provide an accurate true or false result. By including clear naming conventions to my code, I will never have to worry about figuring out a solution to this again.
5. Make your code structure consistent
Whenever I write about my code, I am likely to be biased to my coding methods. However, there is no right or wrong way to code as long as it works and other people can use it. I always try to think about people using my code who may have no idea what it does or how it does it.
By adding a consistent structure to all the code I create, it makes it easier to use and more straightforward to identify bugs when they occur.
Every piece of code I write follows the same structure:
import com.stuff.text.something
import com.atlassian.more.stuff
//-------------------------------------------------------------------------
// This a brief explanation of the script and instructions about
// how to use it.
//-------------------------------------------------------------------------
String myLittleGlobalVariable = "change this value"
String anotherGlobalVariable = "user of the script must change it"
static doSomethingLittle(String localVariableWithProperName) {
//I often use the global variables' value channeled into my local variable.
return someData
}
static getSomeDataFromJira (String localVariableWithProperName) {
//This piece does one tiny thing and nothing else.
}
String catchReturnedData = doSomethingLittle(myLittleGlobalVariable)
write.onScreen(getSomeDataFromJira(catchReturnedData)
Cleanliness is next to godliness
When it comes to code, cleanliness really is next to godliness. Like any skill or craft, practice makes perfect, so working to improve your code every day is key to success.
Using long, descriptive names for your code is the cornerstone of building good software. The building blocks you create one day can be reused the next, standardised naming conventions help to guide you through old scripts and to identify bugs quickly.
When you look at code that is easy to read, there is a good chance you will feel brave enough to alter it, even if you're a novice coder. When you create good clean code, you are showing respect to the people who will use it in the future, and you never know they may even learn something from your work.