Example scripts
To homepage
Jira

Create HTML Table with Issue Details
App in script
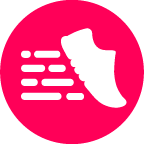
ScriptRunner For Jira
by Adaptavist
Compatibility

Jira
Language |
groovy
import groovy.time.TimeCategory
// Retrieving all bugs
Map<String, Object> bugs = get("/rest/api/3/search?jql=issueType=Bug")
.header('Content-Type', 'application/json')
.header('Accept', 'application/json')
.asObject(Map).body
// Accessing the issues in the payload
def issues = (List<Map<String, Map>>) bugs.issues
def issueData = issues.collect {
// Extracting the issue data we require
String id = it.id
String summary = it.fields.summary.replace('"', '\\"')
String statusChangeDate = it.fields.statuscategorychangedate
String key = it.key
String assignee = ((Map) it.fields.assignee)?.displayName ?: "Unassigned"
String status = (((Map< String, Map>) it.fields.status)?.statusCategory).name ?: "Unknown status"
// Calculating how long an issue has been in it's current status
def timeInStatus = TimeCategory.minus(new Date(), Date.parse("yyy-MM-dd'T'HH:mm:ss.SSSZ", statusChangeDate))
// Creating a formatted string to show the time since the status last changed
def formattedTimeInStatus = ""
if (timeInStatus.days > 0) {
formattedTimeInStatus += "${timeInStatus.days} days, "
}
if (timeInStatus.hours > 0) {
formattedTimeInStatus += "${timeInStatus.hours} hours, "
}
if (timeInStatus.minutes > 0) {
formattedTimeInStatus += "${timeInStatus.minutes} minutes"
}
// Returning an ADF table row for each issue, populated with the data we have extracted
""" {
"type": "tableRow",
"content": [
{
"type": "tableCell",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "${key} / ${id}",
"marks": [
{
"type": "link",
"attrs": {
"href": "${baseUrl}/browse/${key}"
}
}
]
}
]
}
]
},
{
"type": "tableCell",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "${summary}",
"marks": [
{
"type": "em"
}
]
}
]
}
]
},
{
"type": "tableCell",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "${assignee}"
}
]
}
]
},
{
"type": "tableCell",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "${status}"
}
]
}
]
},
{
"type": "tableCell",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "${formattedTimeInStatus}"
}
]
}
]
}
]
}"""
}
// Outputting the entire table, with a row for each issue returned above
new groovy.json.JsonSlurper().parseText("""{
"version": 1,
"type": "doc",
"content": [
{
"type": "table",
"attrs": {
"isNumberColumnEnabled": false,
"layout": "default",
"localId": "2114ce1a-d560-44f6-aca3-8e44e22584a3"
},
"content": [
{
"type": "tableRow",
"content": [
{
"type": "tableHeader",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "Issue Key / Issue ID",
"marks": [
{
"type": "strong"
}
]
}
]
}
]
},
{
"type": "tableHeader",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "Summary",
"marks": [
{
"type": "strong"
}
]
}
]
}
]
},
{
"type": "tableHeader",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "Assignee",
"marks": [
{
"type": "strong"
}
]
}
]
}
]
},
{
"type": "tableHeader",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "Status",
"marks": [
{
"type": "strong"
}
]
}
]
}
]
},
{
"type": "tableHeader",
"attrs": {},
"content": [
{
"type": "paragraph",
"content": [
{
"type": "text",
"text": "Time in Status",
"marks": [
{
"type": "strong"
}
]
}
]
}
]
}
]
},
${issueData.join(",")}
]
}
]
}""")
Having an issue with this script?
Report it here