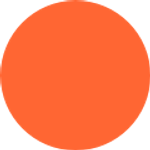
Krisztian Kovacs
Published on 19 July 2018
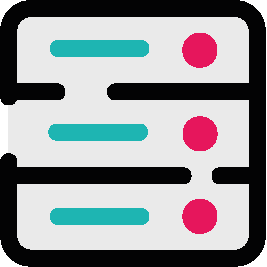
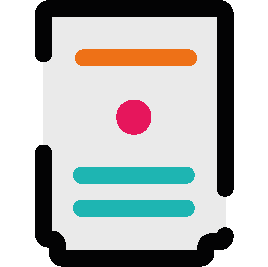
How to integrate everything with Jira
In this step-by-step guide, we help you to create seamless integration links between Jira and other tools.
In other posts, we've looked at how to connect things such as Xmatters to our Jira instance, and now I'd like to go beyond exact solutions and do a more philosophical examination of which parts of your scripts should be changed to create a new integration that connects to something else other than xMatters.
In the end, we must realise that REST API is just a protocol, JSON is a standardised structure, there is nothing Jira or xMatters specific about it. A web application may have a REST API that lets other services send and receive data (to communicate with it).
Connect using REST API
Whenever I'm facing the task of connecting two services, I always ask the same question: can I join them by default? No? How about an add-on or a plugin (same thing, different name) perhaps? Still no?
Oh well, then I guess I'll develop a scripted REST API connection. It would be unthinkable if a web-based piece of software didn't have a REST API. I can connect the entire Atlassian portfolio using only REST API.
Here is how I imagine it:
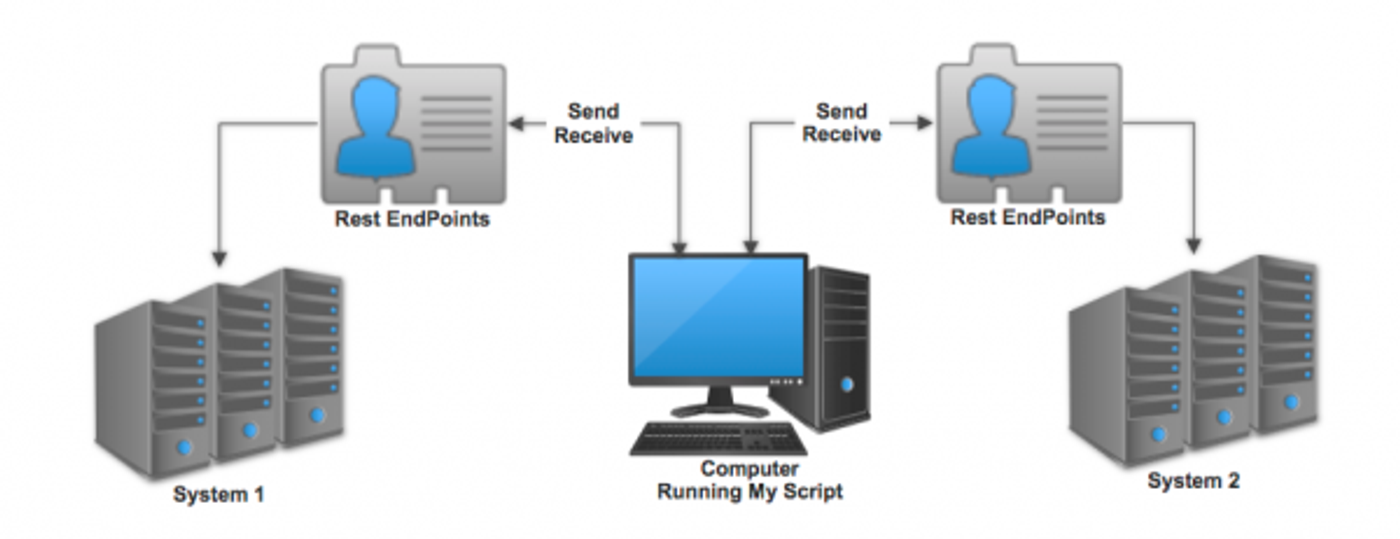
Decisions, decisions, decisions
Do you want to gather data or do you want to send it? In our previous posts, we sent data to xMatters collected from JIRA issues (system and custom fields) but what if you need data from other software? That's not a problem with REST API.
The general idea is the same: you have a URL that you send a request to and in return you'll receive a payload that you can read and (probably) put into custom fields. There are many excellent articles and tutorials on the internet about REST API, so we won't go into detail here, instead we will focus on how best to use REST API.
There is another question to think about: do you need ScriptRunner to call out to another piece of software or can it do it for you? Jira has a myriad of Rest Endpoints ranging from creating an issue to creating an entire project.
In some cases, the best option is to use an add-on to avoid maintaining a solution rather than writing a new script.
Another, quite important, question to ponder is: what do I need to connect via REST API? The answer is quite simple. Most of the time there is a URL with a username and password. Then you either send the data or ask for it. It's quite straightforward and there is always documentation around it. It's a bit like learning the house rules of someone else's home.
Modifying your scripts
To put our theory into practice, you have probably guessed that we need to go in and modify our scripts. But what should we adjust? On the Jira side, not much to be honest. We already have the dialog. Sure, that can be changed to match the new requirements, and the JSON payload will need to change as well. However, this will only change the requirements relating to what you want to send. If you want to send the same data package, you won't need to change anything on the Jira side.
Except maybe the URL, the username and password.
//Global Variables
@Field String xMattersURL =
"https://adaptavist-dev.xmatters.com/api/integration/1/functions/3eb43db8-e0a4-40ff-b1c8-452e89dd4691/triggers"
@Field String xMattersUser = "SOMEUSERNAME"
@Field String xMattersPassword = "SOMEPASSWORD"
Remember the global variables we set up? Well, turns out that's the only thing you'll definitely have to change.
Alternatively you can even rename them, just be careful to rename all the references too.
Developing your scripts
How is it possible to develop scripts easily? you might ask. That's when an Integrated Development Environment (IDE) comes in handy.
The development environment I have set up for myself has the following features:
- Auto-completing class name for groovy (the language of Scriptrunner) and Jira
- Easy refactoring (rename a variable once and it renames it everywhere)
- Syntax highlighting
- Syntax errors
These features do not point at a single IDE, you can choose your own. I'd advise to look around the internet and find the best one to suit your needs. Though, I can give you a helpful hint: IntelliJ has a plugin that can be connected to ScriptRunner.
This way, it's easy for me to rename global variables or method names. All I have to do is to access the context menu when highlighting a variable:
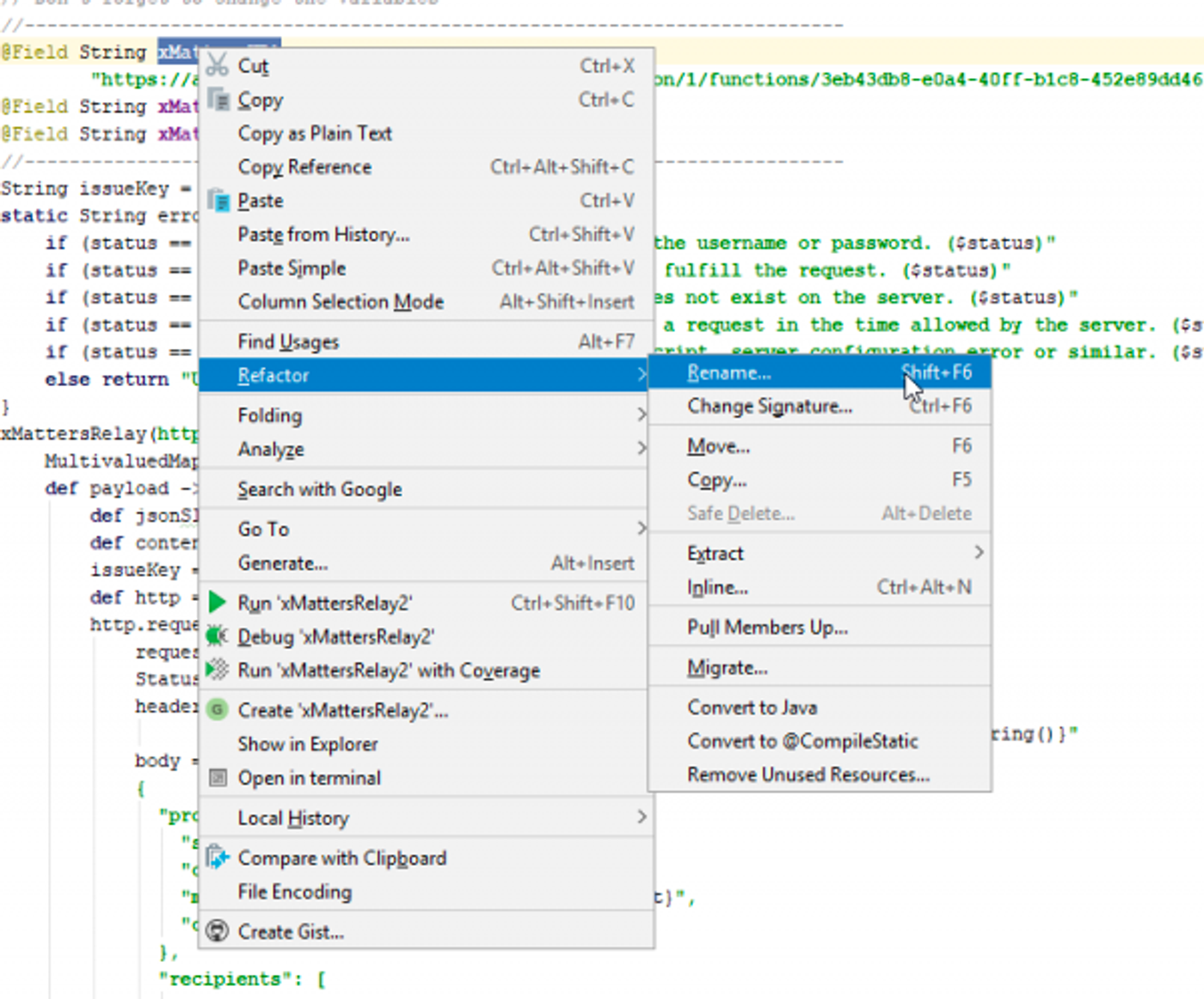
Chat with your developers
The best way to gain more knowledge is to talk to the "dev guys". Most companies have them and while they can be startled easily, they have all the knowledge you'll ever need to get into scripting, and more. There are thousands of YouTube videos, blog posts and tutorials, plus documentation for each piece (Jira and ScriptRunner included) but talking to a human being is always the best option.
They might even help you set up a proper developer environment and give you pointers. There is nothing more rewarding than teaching others how to fish rather than give them the fish!
The other side
We are ready to send our JSON payload. Now that we have fixed all the variables, added everything we wanted on our side, we need to check out the other service, the one receiving the data. Sometimes it's your responsibility to set it up; sometimes it's done by another team. Either way, you should have plenty of information about how the payload should appear, what URL you should send the data to and with what username and password.
Remember: as you already have the data, you only need to rearrange it if needed.
body = """
{
"properties": {
"subject" : "${content.subject}",
"cost": "${content.cost}",
"myLittleSelect": "${content.myLittleSelect}",
"comment": "${content.comment}"
},
"recipients": [
"kkovacs|Work Email"
]
}
"""
The "content.subject" has the data of subject, but is the other side handles it as "subject"? That's what you'll need to find out. If it's, for example, "info" or has some other name, then just rename "subject" to "whatever" (or to "info", it's your call really). The same goes for the other 'variables'.
In case of xMatters, "recipients" needs a list of recipient names with a format of "[name1, name2, nameN]". In our example, I just used my name, but if you followed the previous posts and set up your own xMatters environment you probably used your name. In a different service, you'll probably need something else or maybe even nothing.
xMatters is a notification service, and if you send data to a system that wants to create issues, chances are you won't need the recipient part.
From Russia with love
What if you want to receive data from the other side? Modifying our scripts won't help. You need something else. Maybe there is a Jira add-on for it, or you can use one of Jira's many APIs. You can read more on this here.
You might notice that you have to use a specific URL (officially called URI: Uniform Resource Identifier), a username, a password, and assemble a JSON payload the way "Jira likes it".
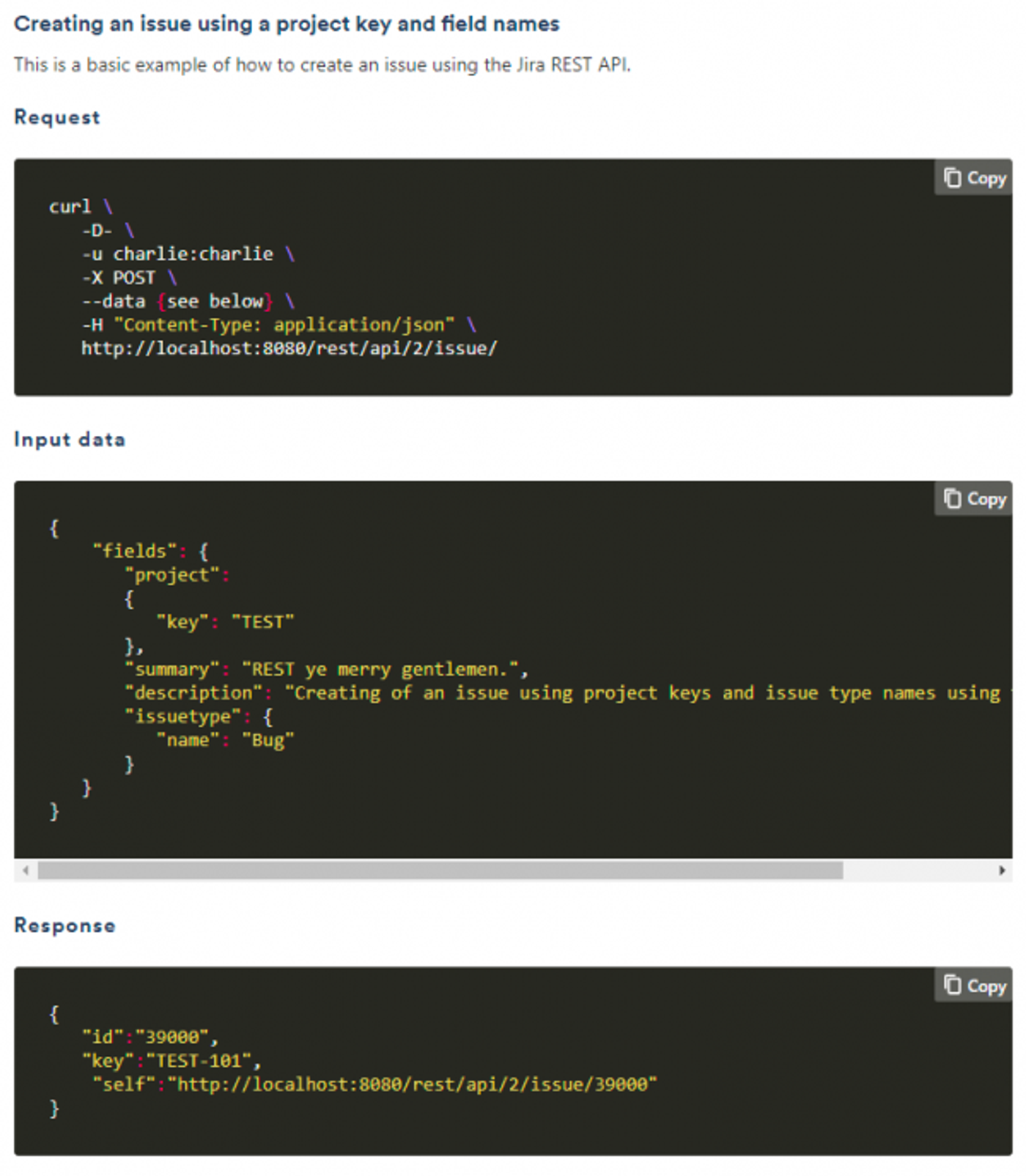
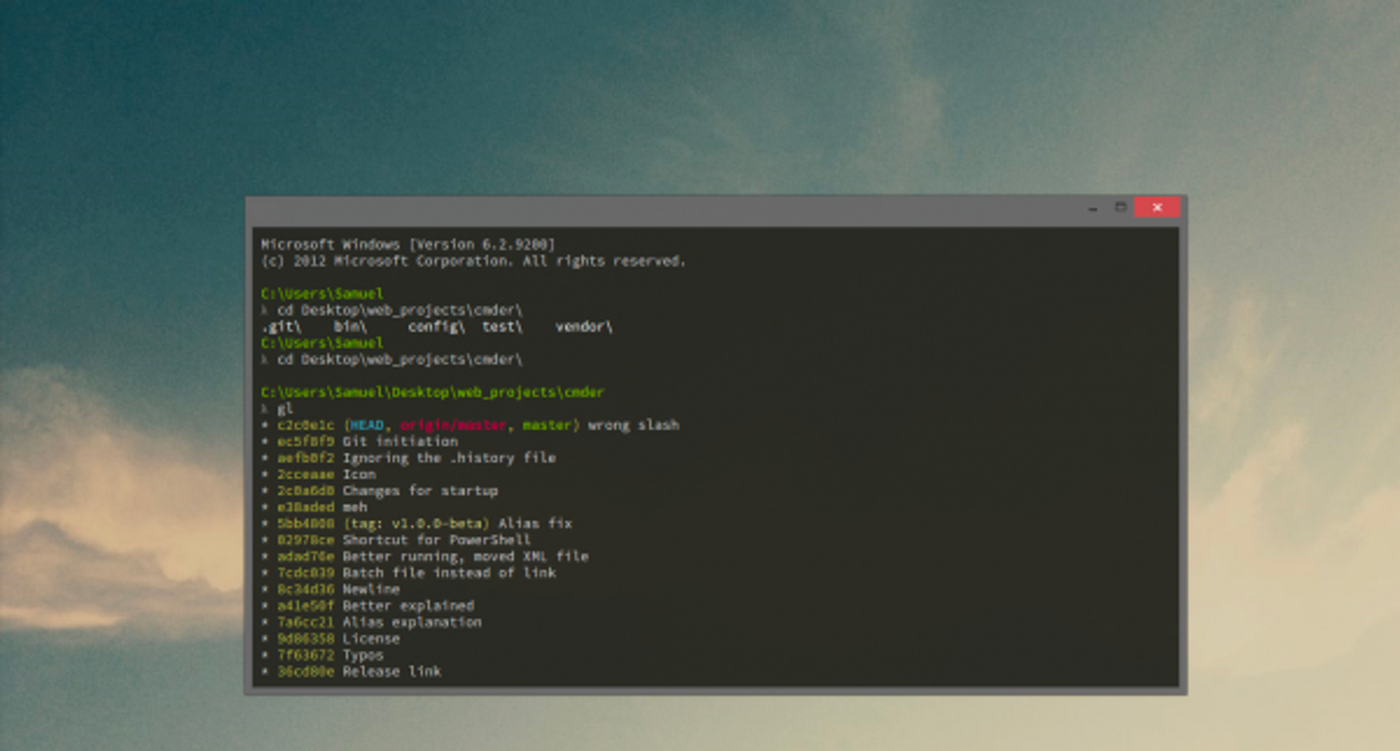
CMDER brings the Linux terminal and other useful stuff right into Windows, such as:
- basic bash commands (like ls, clear, mkdir)
- git (code version control)
- ssh (tunnel into servers remotely)
- scp (copy files to remote servers)
- curl (execute RestAPI)
If in doubt - Google it
I have a file dedicated to methods I regularly use, tailored to my liking and mostly with a "google origin". By that I mean when I need something there are three options available:
- I already have it
- Google has it
- I have to write it
As you can see there is an order to things. I love solving problems, but if someone has already addressed it, there is no need to spend time needlessly working on a solution that already exists.
When I Google something I usually come up with a simple expression, as if I were to start to write a new method, for instance:
- ScriptRunner get Jira base url
- ScriptRunner get custom field data
- Create issue Jira REST API
- Set custom field data REST API
85% of the time the page containing the solution to my problem is an Atlassian Community answer, for the remaining 15% I use Google.
We did it!
xMatters and Jira are connected. Furthermore, we can now connect anything using Rest API (subject to availability). Should you want to go further into how to use ScriptRunner and Rest API, identify the issue and look for solutions.
Remember, there are many ways to solve any problem. We hope this series has helped you get started and to become a bit more comfortable with coding and implementing solutions that go beyond the User Interface.
Coding is ultimately talking to the machine and instructing it to do things. Do it right and you'll be able to sit back and watch your beautiful code do all the hard work for you!
Get started with ScriptRunner
Try ScriptRunner for free with a 30 day trial.