Example scripts
To homepage
Jira

Create Split Custom Field Context for Specific Projects and Issue Types in Jira
App in script
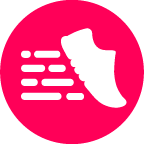
ScriptRunner For Jira
by Adaptavist
Compatibility

Jira
Language |
groovy
// Retrieve the list of all fields from the JIRA API and parse the response as a list
def fields = get("/rest/api/3/field").asObject(List).body
// Find and store the ID of a specific custom field named "My Custom Field"
def myField = fields.find { field ->
field.name == "Select List Field"
}.id
// List of the issue types that the custom field should be available for
// get("/rest/api/3/issuetype").asObject(List).body to get the list of issue types
def issueTypes = ["10005", "10007"] // must be IDs
// Fetch the context configuration for the specific field and parse the response as a map
def context = get("/rest/api/3/field/${myField}/context").asObject(Map).body
// Extract the IDs from all contexts available for the field
def contextIds = context.values*.id
// Initialize an empty list to accumulate all options across different contexts
// Use collect to transform each contextId into a list of options
// Use collectMany to transform each contextId into a list of options and flatten the list
def allOptions = contextIds.collectMany { contextId ->
// Retrieve options for the current field context from the JIRA API
def optionsResponse = get("/rest/api/3/field/${myField}/context/${contextId}/option").asObject(Map).body
// Process the options to remove "id" key from each option and collect into a list
optionsResponse.values.collect { option ->
option.entrySet().findAll { it.key != "id" }.collectEntries()
}
}
// Specify the project names of interest
def projectNames = ["Your project name", "Your second project name"]
// Filter the list of projects to find those that match the specified names
def matchingProjects = Projects.getAllProjects().findAll { it.name in projectNames }
// Extract the project IDs from the matching projects
def projectIds = matchingProjects*.id
// Prepare the data for creating a new context for the field, including description, issue types, name, and project IDs
def contextDescription = "A context used to define the custom field options for bugs."
def contextName = "Your New Context Name"
def contextBody = [
description : contextDescription,
issueTypeIds: issueTypes,
name : contextName,
projectIds : projectIds
]
// Create a new field context by sending a POST request to the JIRA API
def newContext = post("/rest/api/3/field/${myField}/context")
.header("Content-Type", "application/json")
.body(contextBody)
.asObject(Map)
.body
// Extract the ID of the newly created context
def contextId = newContext.id
// Define the body of the request to set options for the new context
def optionsBody = [
options: allOptions
]
// Send a POST request to add options to the newly created context
post("/rest/api/3/field/${myField}/context/${contextId}/option")
.header("Content-Type", "application/json")
.body(optionsBody)
.asObject(Map)
.body
Having an issue with this script?
Report it here